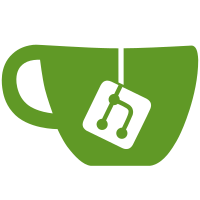
--- type: change message: |- refactor how file changes and hashes are handled, and add tests for hashes This involved a few different changes that all worked together: * Making fileChanged public as ChangedFile, and reorganizing how it's created/used a bit. * Renaming the CommitInterface methods to be clearer, and passing in a `[]ChangedFile` into `ExpectedHash` (previously just `Hash`) rather than trees. * Adding tests for the gen*Hash functions. change_hash: AGCdt2k6FARuf1CBao7zxiuDVMOAdeL5vL8Whnr9KjMu credentials: - type: pgp_signature pub_key_id: 95C46FA6A41148AC body: iQIzBAABAgAdFiEEJ6tQKp6olvZKJ0lwlcRvpqQRSKwFAl6bVGIACgkQlcRvpqQRSKwkmhAAqAuUXKIpr1caE7yuM09udiW51G0TtSe0tLItoZ6ROnSe6lsISZ3hkmOWjSyfeeZFggGIAlK3Uipb42pbSO3Usf0fqDWdNekPLRtTd6qpB65Ye9kDgC3Nz6Z0EhFSMDc6sQzNcisWqAAry6CyEy4ucAm3aK1frSTH/6i/x9DQZXY7WVLM5NewegTr/fatf57Fh6kky7o7yiALeOmQR/oAh7x4AADJgmJMs2aBLsO958GwrNOlQI/RhC83TfoXM7YcTkFv+xz2LucIyBZYYxpM327UTmUk2vuOf3p2OFbiISfIZxFeI3KhpUH05TVXyzohQTnrwyWJDKFGKO8iqxaWHRxdH0INUKsAcBTA8dzTGoRyFF5CUKYX0ZnorlT5NV2HeObmFaDpUHNQKHCcQAAAFoSOGVNNqfGS1IqUNwwZkln+Nzr7YrxmCJ98fDopF0C9ZWhNzNF3+oQbOCIOj3+1kqxsQrcqgK3dwefCL46u244qLDGyqF9s8Aqp4rtrVT+4V2hTB7psdR/EDwu5xZDtXEPEktY+6z0RyYwqxUKBkSklOd+dgmqMYw7YrrNKCW433HjHUEf8Qk/x8CjRANkNYMgOAQqND0pegaAHRJJJbD4xMBrMBO8QlAX5+/ocFfoyEXTKlUuNJXVOh/2TDtOWXhmbVhOBsx7TOhTjUhtAGwE= account: mediocregopher
46 lines
1.4 KiB
Go
46 lines
1.4 KiB
Go
package dehub
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"gopkg.in/src-d/go-git.v4/plumbing"
|
|
"gopkg.in/src-d/go-git.v4/plumbing/filemode"
|
|
"gopkg.in/src-d/go-git.v4/plumbing/object"
|
|
)
|
|
|
|
// ChangedFile describes a single file which has been changed in some way
|
|
// between two object.Trees. If the From fields are empty then the file was
|
|
// created, if the To fields are empty then the file was deleted.
|
|
type ChangedFile struct {
|
|
Path string
|
|
FromMode, ToMode filemode.FileMode
|
|
FromHash, ToHash plumbing.Hash
|
|
}
|
|
|
|
// ChangedFilesBetweenTrees returns the ChangedFile objects which represent the
|
|
// difference between the two given trees.
|
|
func ChangedFilesBetweenTrees(from, to *object.Tree) ([]ChangedFile, error) {
|
|
changes, err := object.DiffTree(from, to)
|
|
if err != nil {
|
|
return nil, fmt.Errorf("could not calculate tree diff: %w", err)
|
|
}
|
|
|
|
changedFiles := make([]ChangedFile, len(changes))
|
|
for i, change := range changes {
|
|
if from := change.From; from.Name != "" {
|
|
changedFiles[i].Path = from.Name
|
|
changedFiles[i].FromMode = from.TreeEntry.Mode
|
|
changedFiles[i].FromHash = from.TreeEntry.Hash
|
|
}
|
|
if to := change.To; to.Name != "" {
|
|
if exPath := changedFiles[i].Path; exPath != "" && exPath != to.Name {
|
|
panic(fmt.Sprintf("unexpected changed path from %q to %q", exPath, to.Name))
|
|
}
|
|
changedFiles[i].Path = to.Name
|
|
changedFiles[i].ToMode = to.TreeEntry.Mode
|
|
changedFiles[i].ToHash = to.TreeEntry.Hash
|
|
}
|
|
}
|
|
return changedFiles, nil
|
|
}
|