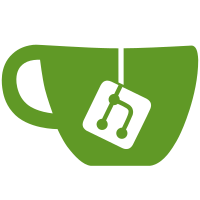
--- type: change message: |- Refactor how commit authorship is formatted This ended up being a loose thread that, when pulled, untangled a bunch of other stuff. Notably the account argument to Repo.Commit is no longer needed, and I added an anon argument to TestSignifierPGP which simplified a number of tests. change_hash: AHMeFpSJb/AoLiELW5pImUiQ+PS0PWibliqcQuFTjC3o credentials: - type: pgp_signature pub_key_id: 95C46FA6A41148AC body: iQIzBAABAgAdFiEEJ6tQKp6olvZKJ0lwlcRvpqQRSKwFAl6TVu8ACgkQlcRvpqQRSKwExw/+OQa++h0ZkFuguU3593D+9P7l8bElMWu+Je40RrDHz4fD0i51UJGcnkk/9fryUWGmWpbCnDv9k7OGztipH/P2HDhuBn+/Dc7X8CcRsRC7/n+o1gd6f1Sc9q4zTgPKkE+ZrE226e8GNVgpZRspbZHa1IwSs/fIkboavnWFowV6SiLColtwYqCfCJXvEP52D+OjKvi4iatRUzoVIOYNHGI4uOufuQRYPZIQRGxgcvUUB/VhjyBB39BV5cHO8oTFmmXH6+eFj4bHWjHsRzp5ferUmsRCdvo2lkoxXkeqN0okyUcwpXQXI7l6BL9OyCxHifIK9G2BaOAsp7A6piwNzaUGk1RIHZpJ69dTfTre1jolOhkGY9lXGAMdSo+ifsFqKj3sXZNjSEJ49riYP98ERnhF1APHN+xL1dkUd8eTTMRh9+C8Bi7twWkUJ2wH5CL1brkpkHIwXOa7jszdeliMK9aZRT7lyxvjCx0uVFTeXbq0RSRb9Oeo+TJhRIu7kLpMKmzX9y/fRaGiPcjr8OD2cfWhACsaVGuU+oXmJXk4uJ+ADfm4IZy7IOEQdr+3Cg33y2mxRq2APwLaGjvA6UFLfar1/nAKK+uTQDF3DssHdLgEfsH2Lu5orc3+FtAblhBiwrN5a732hLceEMkUvQXwOHbZqddkbuqQ6FqHMDIsvBdK6gI= account: mediocregopher
79 lines
3.0 KiB
Go
79 lines
3.0 KiB
Go
package sigcred
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"dehub.dev/src/dehub.git/typeobj"
|
|
)
|
|
|
|
// Credential represents a credential which has been attached to a commit which
|
|
// hopefully will allow it to be included in the main. Exactly one field tagged
|
|
// with "type" should be set.
|
|
type Credential struct {
|
|
PGPSignature *CredentialPGPSignature `type:"pgp_signature"`
|
|
|
|
// AccountID specifies the account which generated this Credential.
|
|
//
|
|
// NOTE that Credentials produced by the direct implementations of
|
|
// SignifierInterface won't fill in this field, unless specifically
|
|
// documented. The SignifierInterface produced by the Interface() method of
|
|
// Signifier _will_ fill this field in, however.
|
|
AccountID string `yaml:"account,omitempty"`
|
|
|
|
// AnonID specifies an identifier for the anonymous user which produced this
|
|
// credential. This field is mutually exclusive with AccountID, and won't be
|
|
// set by any SignifierInterface unless specifically documented.
|
|
AnonID string `yaml:"-"`
|
|
}
|
|
|
|
// MarshalYAML implements the yaml.Marshaler interface.
|
|
func (c Credential) MarshalYAML() (interface{}, error) {
|
|
return typeobj.MarshalYAML(c)
|
|
}
|
|
|
|
// UnmarshalYAML implements the yaml.Unmarshaler interface.
|
|
func (c *Credential) UnmarshalYAML(unmarshal func(interface{}) error) error {
|
|
return typeobj.UnmarshalYAML(c, unmarshal)
|
|
}
|
|
|
|
// ErrNotSelfVerifying is returned from the SelfVerify method of Credential when
|
|
// the Credential does not implement the SelfVerifyingCredential interface. It
|
|
// may also be returned from the SelfVerify method of the
|
|
// SelfVerifyingCredential itself, if the Credential can only self-verify under
|
|
// certain circumstances.
|
|
type ErrNotSelfVerifying struct {
|
|
// Subject is a descriptor of the value which could not be verified. It may
|
|
// be a type name or some other identifying piece of information.
|
|
Subject string
|
|
}
|
|
|
|
func (e ErrNotSelfVerifying) Error() string {
|
|
return fmt.Sprintf("%s cannot verify itself", e.Subject)
|
|
}
|
|
|
|
// SelfVerify will attempt to cast the Credential as a SelfVerifyingCredential,
|
|
// and returns the result of the SelfVerify method being called on it.
|
|
func (c Credential) SelfVerify(data []byte) error {
|
|
el, _, err := typeobj.Element(c)
|
|
if err != nil {
|
|
return err
|
|
} else if selfVerifyingCred, ok := el.(SelfVerifyingCredential); !ok {
|
|
return ErrNotSelfVerifying{Subject: fmt.Sprintf("Credential of type %T", el)}
|
|
} else if err := selfVerifyingCred.SelfVerify(data); err != nil {
|
|
return fmt.Errorf("self-verifying Credential of type %T: %w", el, err)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// SelfVerifyingCredential is one which is able to prove its own authenticity by
|
|
// some means or another. It is not required for a Credential to implement this
|
|
// interface.
|
|
type SelfVerifyingCredential interface {
|
|
// SelfVerify should return nil if the Credential has successfully verified
|
|
// that it has accredited the given data, or an error describing why it
|
|
// could not do so. It may return ErrNotSelfVerifying if the Credential can
|
|
// only self-verify under certain circumstances, and those circumstances are
|
|
// not met.
|
|
SelfVerify(data []byte) error
|
|
}
|