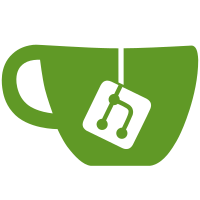
--- type: change message: |- Implement comment commits This ended up requiring a little refactoring here and there, as well as a fix for typeobj after running into a bug. Also made the commit message unmarshaling a bit more durable, after running into a case where the error message that gets produced for an invalid commit is not very helpful. Currently comment commits are not very well tested. The tests for commit objects in general need to be rethought completely, so they better test the hashes as well as message head parsing. change_hash: AO7Cnfcgbuusch969HHVuo8OJCKh+uuiof+qhnmiD5xo credentials: - type: pgp_signature pub_key_id: 95C46FA6A41148AC body: iQIzBAABAgAdFiEEJ6tQKp6olvZKJ0lwlcRvpqQRSKwFAl52W+EACgkQlcRvpqQRSKzFVhAAj3cscGnlSHc9oP20ddKsro1lnJ53Ha22JKKJ3aO8Z33mIX4aXdVTheLFy8gvmtzeVThBzfshCGt3REHD9rYaSfUEUpd3iDwDBYu907h7fDfx9OhxsZhCcbn3k2si9xe2ESDWE4nFuzKq0W9C8HYBhtTDYV6pW3AmJTlLfLeSoH86nemDAsZ/JlwoIXqYbT63h2Y7FZbnPXHzPTo6ZCbf3u6gdOUDG8vifWTXCbxuueSutTYTJ5vHKejhz/WB21GJhvuZnCVrim0T0mVyyE2evCci7SP249tGj2bmSDF/vVD4aurKsyyd8l6Q38MSGYnsNmAMgZPmSzFTrqmY9bjJ8sir8mv0Pn85/ixWNGHVkZ/A9i515YYGNXQfRaHbHU72Yb5mlPWJQhywffhWLxP7HoGn27P2UJ6hh4f6KMiV26nH/4meV6Y2o0623fZzoETHVVp6oks6dMCcYRb5FF54Ogg/uBPeBOvtiIfy8hTwBqGVrzg7Dm2Sl0ystBuN8ZKrxqa24Wl+MukA5bLH/J9himDwl9XQtL+BYyb4vJkJcNqaGkfKyGU4q3Ggt38X71wGMCmYtWMZ9CIx5VC+OJC5B7C1fUOxG67wKG2X9ShY9MVfCtjrdAtnnECjalyPKXwSkbqB1xsdujxmnXhazYJ3Bf//QXc7tJuSuiG6jMjS24I= account: mediocregopher
92 lines
1.9 KiB
Go
92 lines
1.9 KiB
Go
package dehub
|
|
|
|
import (
|
|
"crypto/sha256"
|
|
"encoding/binary"
|
|
"fmt"
|
|
"hash"
|
|
"sort"
|
|
|
|
"gopkg.in/src-d/go-git.v4/plumbing/object"
|
|
)
|
|
|
|
var (
|
|
defaultHashHelperAlgo = sha256.New
|
|
)
|
|
|
|
type hashHelper struct {
|
|
hash.Hash
|
|
varintBuf []byte
|
|
}
|
|
|
|
// if h is nil it then defaultHashHelperAlgo will be used
|
|
func newHashHelper(h hash.Hash) *hashHelper {
|
|
if h == nil {
|
|
h = defaultHashHelperAlgo()
|
|
}
|
|
s := &hashHelper{
|
|
Hash: h,
|
|
varintBuf: make([]byte, binary.MaxVarintLen64),
|
|
}
|
|
return s
|
|
}
|
|
|
|
func (s *hashHelper) sum(prefix []byte) []byte {
|
|
out := make([]byte, len(prefix), len(prefix)+s.Hash.Size())
|
|
copy(out, prefix)
|
|
return s.Hash.Sum(out)
|
|
}
|
|
|
|
func (s *hashHelper) writeUint(i uint64) {
|
|
n := binary.PutUvarint(s.varintBuf, i)
|
|
if _, err := s.Write(s.varintBuf[:n]); err != nil {
|
|
panic(fmt.Sprintf("error writing %x to sha256 sum: %v", s.varintBuf[:n], err))
|
|
}
|
|
}
|
|
|
|
func (s *hashHelper) writeStr(str string) {
|
|
s.writeUint(uint64(len(str)))
|
|
s.Write([]byte(str))
|
|
}
|
|
|
|
func (s *hashHelper) writeTreeDiff(from, to *object.Tree) {
|
|
filesChanged, err := calcDiff(from, to)
|
|
if err != nil {
|
|
panic(err.Error())
|
|
}
|
|
|
|
sort.Slice(filesChanged, func(i, j int) bool {
|
|
return filesChanged[i].path < filesChanged[j].path
|
|
})
|
|
|
|
s.writeUint(uint64(len(filesChanged)))
|
|
for _, fileChanged := range filesChanged {
|
|
s.writeStr(fileChanged.path)
|
|
s.Write(fileChanged.fromMode.Bytes())
|
|
s.Write(fileChanged.fromHash[:])
|
|
s.Write(fileChanged.toMode.Bytes())
|
|
s.Write(fileChanged.toHash[:])
|
|
}
|
|
|
|
}
|
|
|
|
var (
|
|
changeHashVersion = []byte{0}
|
|
commentHashVersion = []byte{0}
|
|
)
|
|
|
|
// if h is nil it then defaultHashHelperAlgo will be used
|
|
func genChangeHash(h hash.Hash, msg string, from, to *object.Tree) []byte {
|
|
s := newHashHelper(h)
|
|
s.writeStr(msg)
|
|
s.writeTreeDiff(from, to)
|
|
return s.sum(changeHashVersion)
|
|
}
|
|
|
|
// if h is nil it then defaultHashHelperAlgo will be used
|
|
func genCommentHash(h hash.Hash, comment string) []byte {
|
|
s := newHashHelper(h)
|
|
s.writeStr(comment)
|
|
return s.sum(commentHashVersion)
|
|
}
|