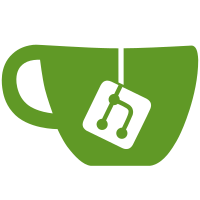
message: |- Rename trunk branch to main branch The term "trunk" is too close to "branch", imo, and is confusing since its inverse term, "thread", isn't related to branch at all. Ultimately I think it's best to leave "branch" as a git specific term, and use "main" and "thread" to denote the different branch types of dehub. change_hash: ANitkSWx+QHnxo/d0zg+lrWdeqx1PHqH2GQ2uvyMYZNa credentials: - type: pgp_signature pub_key_id: 95C46FA6A41148AC body: iQIyBAABAgAdFiEEJ6tQKp6olvZKJ0lwlcRvpqQRSKwFAl5f/ikACgkQlcRvpqQRSKw04Q/4sX9Ylt8vdkhnT24vRqf7uoO2WsRCs4uHvr3tW5V735o3ReKE4KWT9crIKSSn92vkEaFdNAr8+9M7CMfa3qKLAd0kVbGIDiZ775+4jw/Px+nyORSkl17F5gji3m3kM6rnvqYtg5r1NvxuIeuqNK8TGojtlAsf1hN6inrB6S7MEAgw/wYRMQjV7ohYFkeMMLzaN+Q1YA4LolfnM4JbsIwcFMHC1jxDe6is22lUxR1XqTEzJqpIQLLCtY4Ds6LECqm0cFRlXic1ldcYqHxZvWLks8MdNtkMq0FqwEUtr07IqWUAyWcMsmKkReSbTfqPlizJOZ9J4c+8p5FIkRNl+TuJnfB4oBNANMmEHeeHNY3a5pfeJFOyfzAq7LfOwYBMfWcNvX4kuKfAKhSI55Snb6vK4ChRget3VruOZaFIjfAxWQX5QVcDufh3EJvuCJ1lJKKxdqIHQO95hvJ4cMgz1+pxPnQabEkFlk0Q+qFYS4u1S89y5lWxc5GIRaZX6X43/r74rB027LN9eqsovrJ0MLFHRH3QagS9YxDUsVWnv1Y/8TvUn65QRIsHbmrF1ESYdjycROh1bhTJLwwqrSwQjm5HhquvJ93DzUNgRZDLRXwDGL2raJ7oeNL+6E2fl8ahPntmvdVFWtXsMNK93/IKkW3brxUoBkWt5rzKqE4TRhWjDw== account: mediocregopher
179 lines
4.2 KiB
Go
179 lines
4.2 KiB
Go
package accessctl
|
|
|
|
import (
|
|
"reflect"
|
|
"testing"
|
|
|
|
"github.com/davecgh/go-spew/spew"
|
|
)
|
|
|
|
func normalizeResult(res MatchResult) MatchResult {
|
|
if len(res.ChangeAccessControls) == 0 {
|
|
res.ChangeAccessControls = nil
|
|
}
|
|
return res
|
|
}
|
|
|
|
func TestMatch(t *testing.T) {
|
|
secondCond := Condition{
|
|
Signature: &ConditionSignature{
|
|
AnyAccount: true,
|
|
Count: "2",
|
|
},
|
|
}
|
|
|
|
tests := []struct {
|
|
descr string
|
|
|
|
branchACs []BranchAccessControl
|
|
interactions MatchInteractions
|
|
result MatchResult
|
|
}{
|
|
{
|
|
descr: "empty input empty result",
|
|
result: MatchResult{
|
|
BranchPattern: "**",
|
|
},
|
|
},
|
|
{
|
|
descr: "empty access controls",
|
|
interactions: MatchInteractions{
|
|
Branch: "main",
|
|
FilePathsChanged: []string{"foo", "bar"},
|
|
},
|
|
result: MatchResult{
|
|
BranchPattern: "main",
|
|
ChangeAccessControls: []MatchedChangeAccessControl{
|
|
{
|
|
ChangeAccessControl: DefaultChangeAccessControl,
|
|
FilePaths: []string{"foo", "bar"},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
descr: "empty filesPathsChanged",
|
|
branchACs: DefaultBranchAccessControls,
|
|
interactions: MatchInteractions{Branch: "main"},
|
|
result: MatchResult{BranchPattern: "main"},
|
|
},
|
|
{
|
|
descr: "no matching branch patterns",
|
|
branchACs: []BranchAccessControl{{
|
|
BranchPattern: "dunk",
|
|
ChangeAccessControls: []ChangeAccessControl{{
|
|
FilePathPattern: "**",
|
|
Condition: secondCond,
|
|
}},
|
|
}},
|
|
interactions: MatchInteractions{
|
|
Branch: "crunk",
|
|
FilePathsChanged: []string{"foo"},
|
|
},
|
|
result: MatchResult{
|
|
BranchPattern: "**",
|
|
ChangeAccessControls: []MatchedChangeAccessControl{{
|
|
ChangeAccessControl: DefaultChangeAccessControl,
|
|
FilePaths: []string{"foo"},
|
|
}},
|
|
},
|
|
},
|
|
{
|
|
descr: "no matching files",
|
|
branchACs: []BranchAccessControl{{
|
|
BranchPattern: "main",
|
|
ChangeAccessControls: []ChangeAccessControl{{
|
|
FilePathPattern: "boo",
|
|
Condition: secondCond,
|
|
}},
|
|
}},
|
|
interactions: MatchInteractions{
|
|
Branch: "main",
|
|
FilePathsChanged: []string{"foo"},
|
|
},
|
|
result: MatchResult{
|
|
BranchPattern: "main",
|
|
ChangeAccessControls: []MatchedChangeAccessControl{{
|
|
ChangeAccessControl: DefaultChangeAccessControl,
|
|
FilePaths: []string{"foo"},
|
|
}},
|
|
},
|
|
},
|
|
{
|
|
descr: "branch pattern precedent",
|
|
branchACs: []BranchAccessControl{
|
|
{
|
|
BranchPattern: "main",
|
|
ChangeAccessControls: []ChangeAccessControl{{
|
|
FilePathPattern: "foo",
|
|
Condition: secondCond,
|
|
}},
|
|
},
|
|
{
|
|
BranchPattern: "**",
|
|
ChangeAccessControls: []ChangeAccessControl{
|
|
DefaultChangeAccessControl,
|
|
},
|
|
},
|
|
},
|
|
interactions: MatchInteractions{
|
|
Branch: "main",
|
|
FilePathsChanged: []string{"foo"},
|
|
},
|
|
result: MatchResult{
|
|
BranchPattern: "main",
|
|
ChangeAccessControls: []MatchedChangeAccessControl{{
|
|
ChangeAccessControl: ChangeAccessControl{
|
|
FilePathPattern: "foo",
|
|
Condition: secondCond,
|
|
},
|
|
FilePaths: []string{"foo"},
|
|
}},
|
|
},
|
|
},
|
|
{
|
|
descr: "multiple files matching FilePathPatterns",
|
|
branchACs: []BranchAccessControl{{
|
|
BranchPattern: "main",
|
|
ChangeAccessControls: []ChangeAccessControl{{
|
|
FilePathPattern: "foo*",
|
|
Condition: secondCond,
|
|
}},
|
|
}},
|
|
interactions: MatchInteractions{
|
|
Branch: "main",
|
|
FilePathsChanged: []string{"foo_a", "bar", "foo_b"},
|
|
},
|
|
result: MatchResult{
|
|
BranchPattern: "main",
|
|
ChangeAccessControls: []MatchedChangeAccessControl{
|
|
{
|
|
ChangeAccessControl: DefaultChangeAccessControl,
|
|
FilePaths: []string{"bar"},
|
|
},
|
|
{
|
|
ChangeAccessControl: ChangeAccessControl{
|
|
FilePathPattern: "foo*",
|
|
Condition: secondCond,
|
|
},
|
|
FilePaths: []string{"foo_a", "foo_b"},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
}
|
|
|
|
for _, test := range tests {
|
|
t.Run(test.descr, func(t *testing.T) {
|
|
res, err := Match(test.branchACs, test.interactions)
|
|
if err != nil {
|
|
t.Fatalf("error matching: %v", err)
|
|
}
|
|
res, expRes := normalizeResult(res), normalizeResult(test.result)
|
|
if !reflect.DeepEqual(res, expRes) {
|
|
t.Fatalf("expected:%s\ngot: %s", spew.Sdump(expRes), spew.Sdump(res))
|
|
}
|
|
})
|
|
}
|
|
}
|