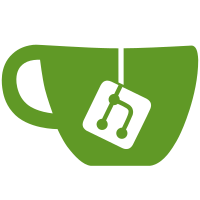
message: Initial commit, can create master commit and verify previous master commits change_hash: ADgeVBdfi1hA0TTDrBIkYHaQQYoxZaInZz1p/BAH35Ng credentials: - type: pgp_signature pub_key_id: 95C46FA6A41148AC body: iQIzBAABAgAdFiEEJ6tQKp6olvZKJ0lwlcRvpqQRSKwFAl5IbRgACgkQlcRvpqQRSKzWjg/+P0a3einWQ8wFUe05qXUbmMQ4K86Oa4I85pF6kubZlFy/UbcjiPnTPRMKAhmGZi4WCz1sW1F2al4qKvtq3nvn6+hZY8dj0SjPgGG2lkMMLEVy1hjsO7d9S9ZEfUv0cHOcvkphgVQk+InkegBXvFS45mwKQLDOiW5tPcTFDHTHBmC/nlCV/sKCrZEmQGU7KaELJKOf26LSY2zXe6fbVCa8njpIycYS7Wulu2OODcI5n6Ye2U6DvxN6MvuNvziyX7VMePS1xEdJYpltsNMhSkMMGLU7dovxbrhD617uwOsm1847YX9HTJ3Ixs+M0yobHmz8ob4OBcZx8r3AoiyDo+HNMmAZ96ue8pPHmI+2O9jEmbmbH61yq4crhUVAP8PncSTdq0tiYKj/zaSTJ8CT2W0uicX/3v9EtIFn0thqe/qZzHh6upixvpXDpNjZZ5SxiVm8MITnWzInQRbo9yvFsfgd7LqMGKZeGv5q5rgNTRM4fwGrJDuslwj8V2B4uw1ofPncL+LHmXArXWiewvvJFU2uRpfvsl+u4is2dl2SGVpe7ixm+a088gllOQCMRgLbuaN8dQ/eqdkfdxUg+SYQlx6vykrdJOSQrs9zaX/JuxnaNBTi/yLY1FqFXaXBGID6qX1cnPilw+J6vEZYt1MBtzXX+UEjHyVowIhMRsnts6Wq3Z8= account: mediocregopher
111 lines
2.4 KiB
Go
111 lines
2.4 KiB
Go
package accessctl
|
|
|
|
import (
|
|
"dehub/sigcred"
|
|
"reflect"
|
|
"testing"
|
|
)
|
|
|
|
func TestConditionSignatureSatisfied(t *testing.T) {
|
|
tests := []struct {
|
|
descr string
|
|
cond ConditionSignature
|
|
credAccountIDs []string
|
|
err error
|
|
}{
|
|
{
|
|
descr: "no cred accounts",
|
|
cond: ConditionSignature{
|
|
AnyAccount: true,
|
|
Count: "1",
|
|
},
|
|
err: ErrConditionSignatureUnsatisfied{
|
|
TargetNumAccounts: 1,
|
|
NumAccounts: 0,
|
|
},
|
|
},
|
|
{
|
|
descr: "one cred account",
|
|
cond: ConditionSignature{
|
|
AnyAccount: true,
|
|
Count: "1",
|
|
},
|
|
credAccountIDs: []string{"foo"},
|
|
},
|
|
{
|
|
descr: "one matching cred account",
|
|
cond: ConditionSignature{
|
|
AccountIDs: []string{"foo", "bar"},
|
|
Count: "1",
|
|
},
|
|
credAccountIDs: []string{"foo"},
|
|
},
|
|
{
|
|
descr: "no matching cred account",
|
|
cond: ConditionSignature{
|
|
AccountIDs: []string{"foo", "bar"},
|
|
Count: "1",
|
|
},
|
|
credAccountIDs: []string{"baz"},
|
|
err: ErrConditionSignatureUnsatisfied{
|
|
TargetNumAccounts: 1,
|
|
NumAccounts: 0,
|
|
},
|
|
},
|
|
{
|
|
descr: "two matching cred accounts",
|
|
cond: ConditionSignature{
|
|
AccountIDs: []string{"foo", "bar"},
|
|
Count: "2",
|
|
},
|
|
credAccountIDs: []string{"foo", "bar"},
|
|
},
|
|
{
|
|
descr: "one matching cred account, missing one",
|
|
cond: ConditionSignature{
|
|
AccountIDs: []string{"foo", "bar"},
|
|
Count: "2",
|
|
},
|
|
credAccountIDs: []string{"foo", "baz"},
|
|
err: ErrConditionSignatureUnsatisfied{
|
|
TargetNumAccounts: 2,
|
|
NumAccounts: 1,
|
|
},
|
|
},
|
|
{
|
|
descr: "50 percent matching cred accounts",
|
|
cond: ConditionSignature{
|
|
AccountIDs: []string{"foo", "bar", "baz"},
|
|
Count: "50%",
|
|
},
|
|
credAccountIDs: []string{"foo", "bar"},
|
|
},
|
|
{
|
|
descr: "not 50 percent matching cred accounts",
|
|
cond: ConditionSignature{
|
|
AccountIDs: []string{"foo", "bar", "baz"},
|
|
Count: "50%",
|
|
},
|
|
credAccountIDs: []string{"foo"},
|
|
err: ErrConditionSignatureUnsatisfied{
|
|
TargetNumAccounts: 2,
|
|
NumAccounts: 1,
|
|
},
|
|
},
|
|
}
|
|
|
|
for _, test := range tests {
|
|
t.Run(test.descr, func(t *testing.T) {
|
|
creds := make([]sigcred.Credential, len(test.credAccountIDs))
|
|
for i := range test.credAccountIDs {
|
|
creds[i].AccountID = test.credAccountIDs[i]
|
|
}
|
|
|
|
err := test.cond.Satisfied(creds)
|
|
if !reflect.DeepEqual(err, test.err) {
|
|
t.Fatalf("Satisfied returned %#v\nexpected %#v", err, test.err)
|
|
}
|
|
})
|
|
}
|
|
}
|