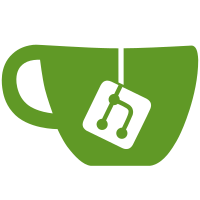
--- type: change message: |- completely refactor accessctl (again) This time it's using an actual access control list system, rather than whatever it was doing before. The new system uses a Filter type, rather than Condition, to decide which acl element should have its action (allow or deny) applied. This makes testing way easier, since all the different matching conditions are now individual filters, and so are tested individually. change_hash: AFgN0hormIlO0VWkLKnAdSDZeVRbh0Wj8LLXOMVQEK+L credentials: - type: pgp_signature pub_key_id: 95C46FA6A41148AC body: iQIzBAABAgAdFiEEJ6tQKp6olvZKJ0lwlcRvpqQRSKwFAl5yoi8ACgkQlcRvpqQRSKwo/g//QkSA80APnPtwcibNeaoUwduH1Xim8pmi5JScKGsOypYkE0Iy+fO3fRNz4r7Tm6qNn7O04fDsiXlxPojxn7+NDFCQArVgoJk3jVTRDBW7LpahWJsYPP1SBjGtaR9o0bOpclXblTMIcteTkLM94AeASqLaEY8StO+5PX/82AkFRQ8E6m9R2HCmgwbBhqwWp8936x8ekMFbSSi0TMIyV4rpd0wj4mjvjjBwa3ArGmH/ynwabPCFuuqMT6996N1zoDn5EqZA5jGrf+Q7rxsI6t1bOnLmg9NGMQYRaZLAVZrp5P6G5XR3et4Gz/2AphAEgYJM3yLbEjZW6Daa77CgTNHXde7gCaWqyfcKlVGPi29/O+2IXhpjwxHwGpsBgEdX9227zapL+jwSAOdUVj8n6C8I8BGqpT7rTwA53yxlbSwXlkttvAn/lGT5X4lK74YfkzMXMEBZKzsb/dQEPyP2Y+AG6z2D4Bs/4szsCiUXF9aG2Yx1o45lVXTTdPUNLIsnhBjM7usbQRg8i5kC+OC9AVCi8E+lf0/Qgp0cUb6QLH47bHvDTH7UluY1bgSLZy+Zjaisvl3a0aK/UspywWN/fFgOrz2cDw232n8IC+Zi4LSKm7dXDRFbC1JNzrwAPP1ifboOrltwKroOsDNaVGhX8ABahNjmrUO4JgE7gvX+zxXb+/I= account: mediocregopher
49 lines
1.4 KiB
Go
49 lines
1.4 KiB
Go
package dehub
|
|
|
|
import (
|
|
"dehub/sigcred"
|
|
"testing"
|
|
)
|
|
|
|
func TestConfigChange(t *testing.T) {
|
|
h := newHarness(t)
|
|
|
|
var gitCommits []GitCommit
|
|
|
|
// commit the initial staged changes, which merely include the config and
|
|
// public key
|
|
gitCommit := h.changeCommit("commit configuration", h.cfg.Accounts[0].ID, h.sig)
|
|
gitCommits = append(gitCommits, gitCommit)
|
|
|
|
// create a new account and add it to the configuration. That commit should
|
|
// not be verifiable, though
|
|
newSig, newPubKeyBody := sigcred.SignifierPGPTmp("toot", h.rand)
|
|
h.cfg.Accounts = append(h.cfg.Accounts, Account{
|
|
ID: "toot",
|
|
Signifiers: []sigcred.Signifier{{PGPPublicKey: &sigcred.SignifierPGP{
|
|
Body: string(newPubKeyBody),
|
|
}}},
|
|
})
|
|
|
|
h.stageCfg()
|
|
badCommit, err := h.repo.NewCommitChange("add toot user")
|
|
if err != nil {
|
|
t.Fatalf("creating CommitChange: %v", err)
|
|
}
|
|
h.tryCommit(false, badCommit, h.cfg.Accounts[1].ID, newSig)
|
|
|
|
// now add with the root user, this should work.
|
|
h.stageCfg()
|
|
gitCommit = h.changeCommit("add toot user", h.cfg.Accounts[0].ID, h.sig)
|
|
gitCommits = append(gitCommits, gitCommit)
|
|
|
|
// _now_ the toot user should be able to do things.
|
|
h.stage(map[string]string{"foo/bar": "what a cool file"})
|
|
gitCommit = h.changeCommit("add a cool file", h.cfg.Accounts[1].ID, newSig)
|
|
gitCommits = append(gitCommits, gitCommit)
|
|
|
|
if err := h.repo.VerifyCommits(MainRefName, gitCommits); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
}
|